According to The State of the Developer Ecosystem 2019, 65% of PHP developers relies on var_dump()
, dd()
, etc… to debug their applications, while only the 35% uses a proper debugger. [1]
Printing the content of a variable is effective, but not really efficient when it comes to follow its lifecycle in an application.
Debugging is an important skill for a developer, but setting up the correct environment may be tedious. I will try to address this in this post.
Installing xDebug
You should first check that you don’t have xDebug already installed on your container.
Access your container (i.e. docker exec -it your-cantainer bash
) and run php -v
.
If your output shows that xDebug is installed, as below, you skip to the next section.
PHP 7.4.11 (cli) (built: Oct 6 2020 10:35:51) ( NTS )
Copyright (c) The PHP Group
Zend Engine v3.4.0, Copyright (c) Zend Technologies
...
with Xdebug v2.9.6, Copyright (c) 2002-2020, by Derick Rethans
Otherwise, edit your Dockerfile to install php-xdebug
from the package manager.
This is an example with Debian:
RUN apt-get update \
&& apt-get -y --no-install-recommends install php-xdebug \
&& apt-get clean; rm -rf /var/lib/apt/lists/* /tmp/* /var/tmp/* /usr/share/doc/*
Rebuilding the container and running php -v
again should now output the version of xDebug.
Setting up xDebug
In a directory of your preference in your project (that could be the one were you have your Dockerfile), create a file named ext-xdebug.ini
with this content:
zend_extension=xdebug.so
[Xdebug]
xdebug.remote_enable=true
xdebug.remote_port=9001
xdebug.remote_host=host.docker.internal
We are configuring xDebug to receive remote connections on port 9001
on the internal host of Docker.
In docker-compose.yaml
, configure the file to be copied in the right directory:
version: "3.1"
services:
php-fpm:
build: docker/php
...
volumes:
...
- ./docker/php/ext-xdebug.ini:/etc/php/7.4/fpm/conf.d/ext-xdebug.ini
Don’t forget to change the path accordingly with your PHP version
Rebuild the container again.
Preparing PHPStorm
This guide uses PhpStorm 2020.2.3 for MacOS.
Setting up the CLI interpreter
Open “Preferences
” and access “PHP
” under “Languages & Frameworks
” and click the three dots on the right of “CLI Interpreter
“, where it says “<no interpreter>
“.
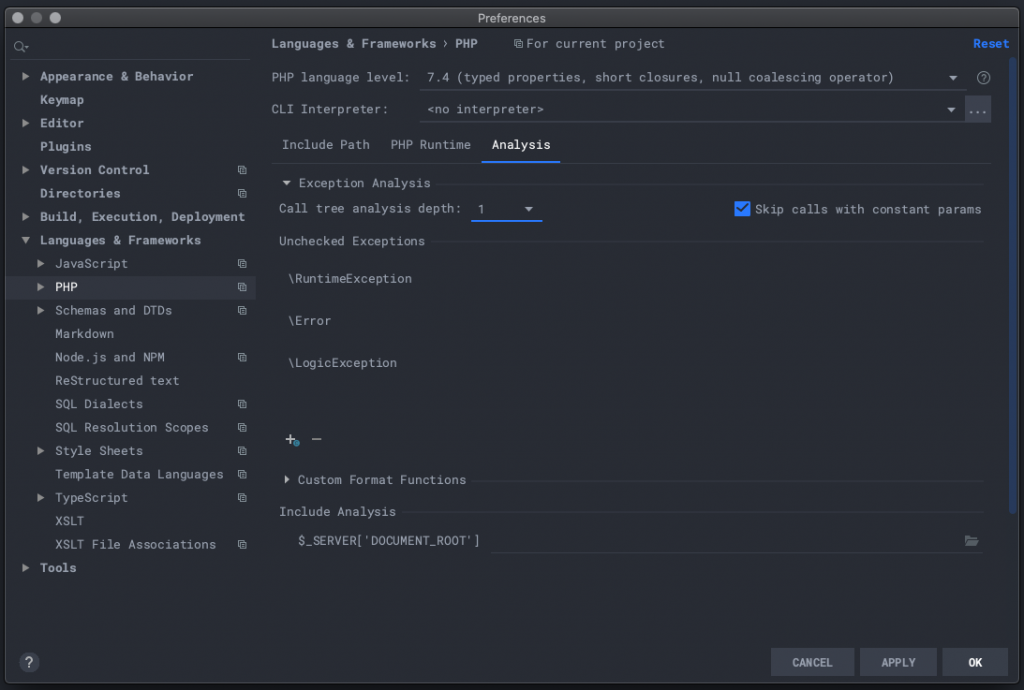
Doing this will open up a new windows that will eventually list all the interpreters you have configured. Click the “+
” sign and select “From Docker, Vagrant, VM, WSL, Remote...
“
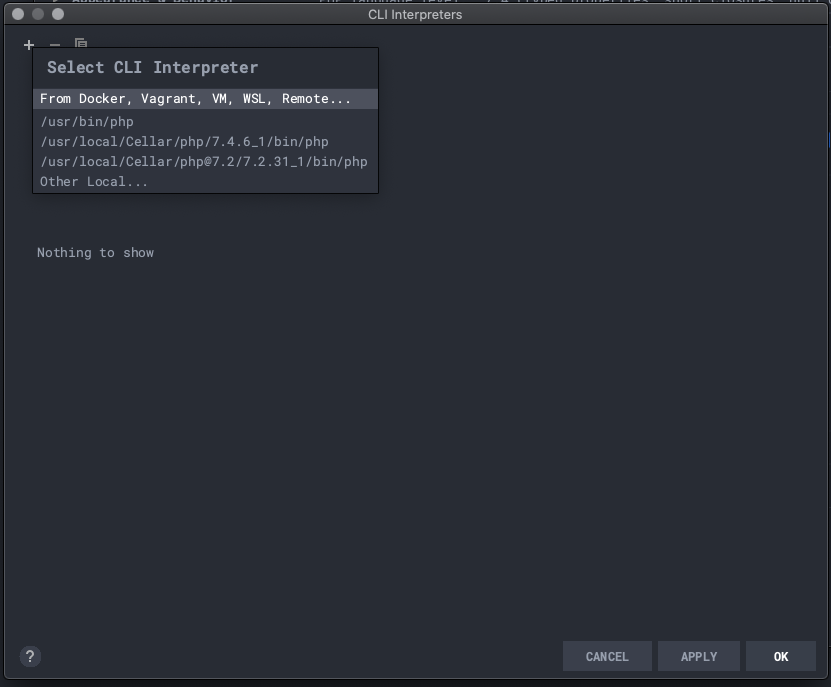
A new window will open up. Select “Docker
” and choose the image of your PHP container.
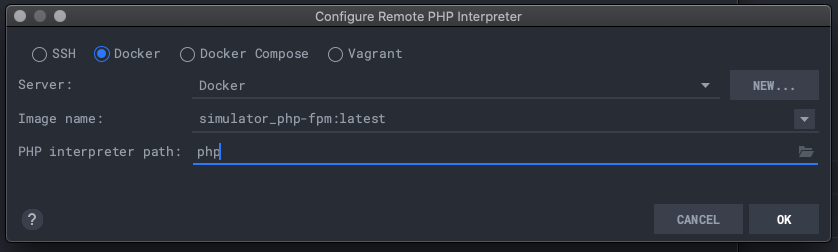
Clicking OK
will close the window and we can see that xDebug is correctly shown for this interpreter.
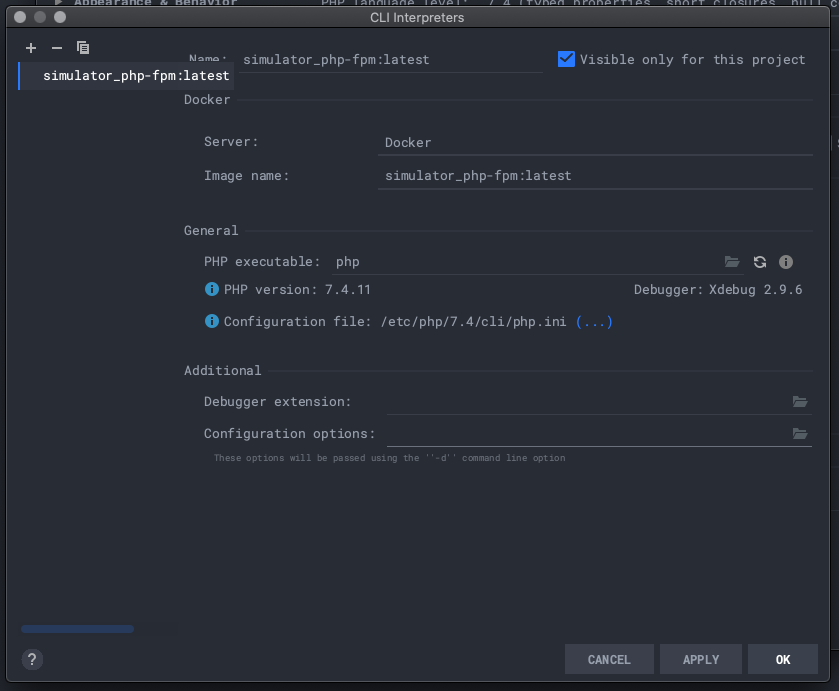
Clicking OK
again will close the window and set up the interpreter for us.
Adding a configuration
Now we have to create a new configuration for our project.
From the window that will pop up, click the “+
” sign and select “PHP
Script`”.
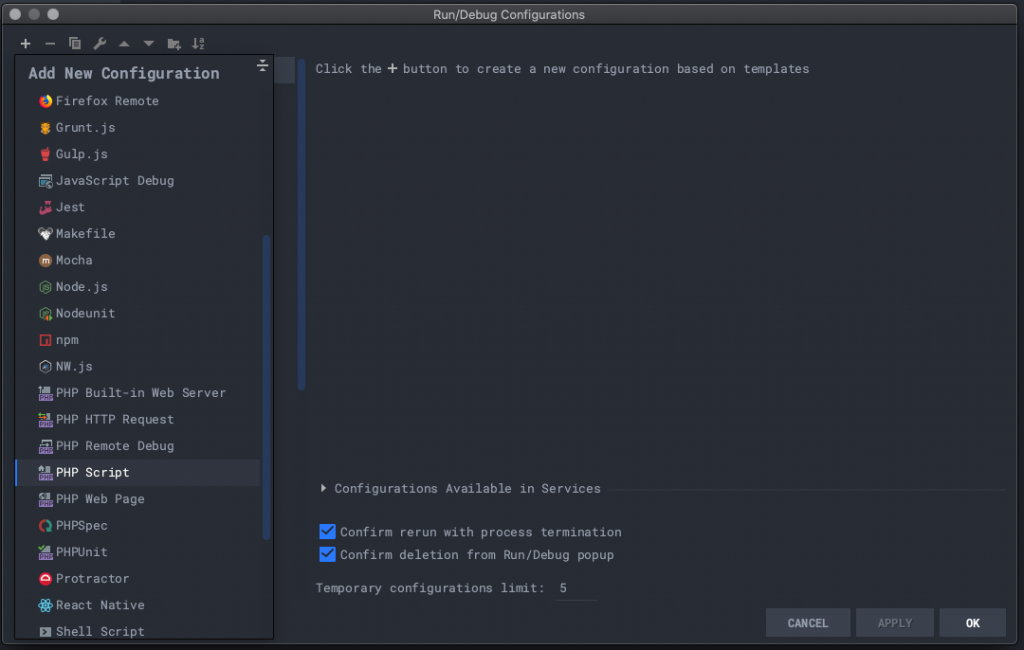
Give a name of your choice and select the interpreter we created earlier from the list of interpreters.
Specify the name of the file you want to run and any arguments required.
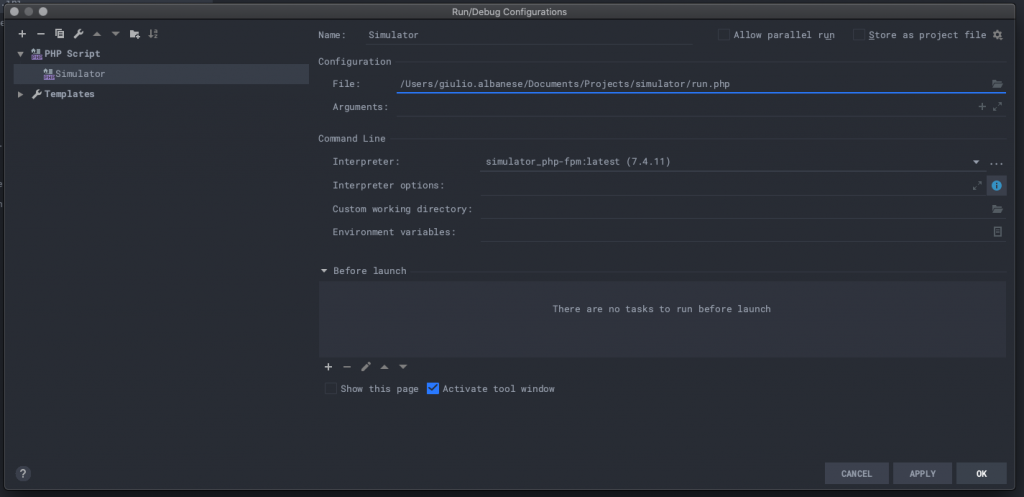
Starting to debug
Open your code and set some breakpoints by clicking between the line number and the code.

Now start the script with the configuration by clicking the 🐞 icon in the toolbar near the ▶️ button.
The script will run and the debugger will stop the execution on the first breakpoint, showing you all the variables in the context you currently are.
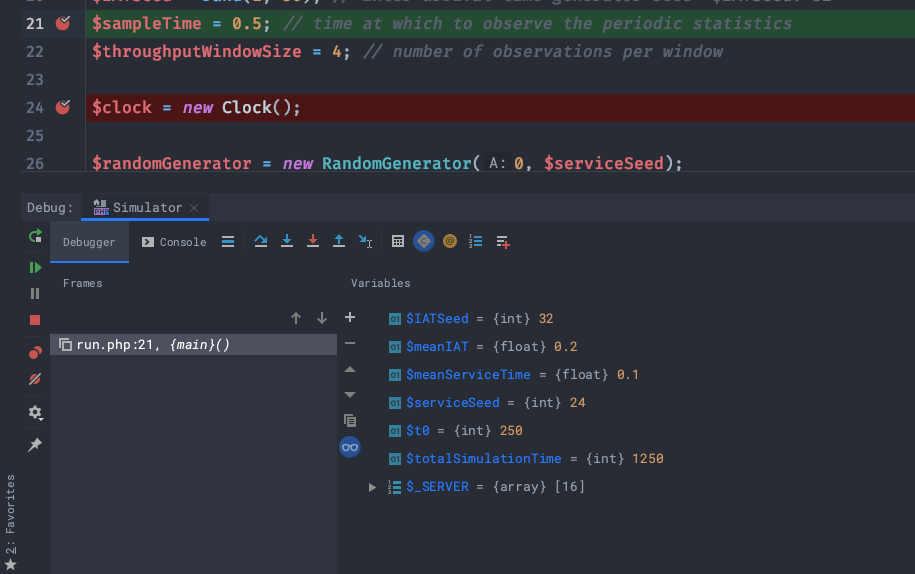
The buttons at the top and the left of the debugger window will allow you to control the execution of the script. You can jump to the next breakpoint, go line by line, jump over a line, step inside a function, etc…
Thank you for getting this far! ❤️
If this guide was helpful to you or you have any doubt, consider tweeting me.